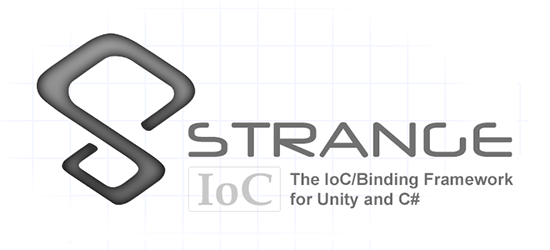
Glossary of Terms
These are terms that every engineer working with StrangeIoC should understand. None of these is peculiar to Strange...they're just general-purpose engineering terms with which you're probably already familiar. But if perchance you run across a term in the docs that you don't know, check here. If you want to go deeper, they're all defined in more detail on Wikipedia.
I've also made note wherever we perhaps play a little loosely with a term in StrangeIoC.
MVC / MVCS
Model-View-Controller (MVC) or Model-View-Controller-Servce (MVCS) is a design pattern for implementing user-facing applications. It breaks the application into 3 (or 4) distinct areas: (1) Models hold application state; (2) Controllers handle logic; (3) Views represent everything you see, hear or interact with; and (4) Services handle anything outside the application (such as communications with a device or the Web).
In Strange, we recommend using the MVCSContext as a way of setting up the overall application, while the main game operates within Unity's entity-based Update loop.
Command Pattern
The Command Pattern is a design pattern in which an invoking class triggers a result that performs a specific task. Strange uses Commands in a very literal way: fired events or Signals result in creation of a Command instance that performs the requested task. Strange allows you to bind an event or Signal to one Command or many, so that work is done in a highly abstract and reusable fashion. Commands largely represent the 'Controller' aspect of the MVCS pattern.
Composition
Composition is simply the process of joining parts into a useful whole. Both Entity systems (like Unity) and Injection systems (like Strange) allow you to build highly complex instances out of relatively simple building blocks.
Object Composition - Wikipedia
Controller
Controller is the 'C' in MVC / MVCS. Controllers send instructions around the application and perform logic. Essentially, Controllers manipulate Models and Services, and return information to Views.
Most Controller code in Strange is handled in Commands.
Coupling
See Dependency
Dependency
Dependency (or coupling) is the degree to which one class cannot function without the assistance of another. Tightly coupled systems tend to be inflexible and to break easily. Minimizing dependencies makes code more robust, and is the central goal of StrangeIoC.
Dependency/Coupling - Wikipedia
Dependency Injection
In Object-Oriented Programming, Dependency Injection is a strategy for providing an Object's required dependencies with a minimum of entangling complications. It is closely related to the Service Locator and Factory design patterns. In dependency injection, a central agency (the Injector) provides a client class' internal dependencies at runtime. The client defines its dependencies as broadly as possible. This enables flexibility, because the dependency can be swapped out for any other dependency that satisfies that broad definition. It encourages testability, since broadly-defined dependencies can be replaced by 'mocks' for unit testing, and well-tested code is less apt to break unexpectedly.
Dependency Injection - Wikipedia
Design Pattern
Design patterns are a general concept in programming that relates to common problems with well-understood solutions. The patterns are given names which allow engineers to discuss a complex problem and the proposed solution with a simple word or phrase (therefore "Adapter" or "Facade" rather than "this class doesn't conform to our needs quite the way we want to use it, so we'll write a class that includes an instance of the original class, altering and/or combining that class's methods to conform with what we really want").
Not every pattern is considered 'good'. Most patterns do a good job of solving a problem, but many solve one problem while creating another (sometimes called an 'anti-pattern').
All engineers should understand the principle of design patterns as they relate to their own discipline. For example, there are many common design patterns in object-oriented application and game design. In Strange, we talk a lot about specific patterns, including: MVCS, Service Locator, Factory, Command, Singleton, and Observer. Each of these patterns is described briefly in this glossary. For a fuller description of these and other patterns, check Wikipedia.
Entity / Entity System
Entity systems are extremely composition-oriented programming structures. An entity itself is nothing but a container and an identifier, but it can be added to such that it begins to have useful behavior. In Unity, the GameObject may (nearly) be thought of as such an entity. By default, all GameObjects get a Transform...so it's not quite 'nothing'...but the analogy works in that the developer starts attaching Monobehaviours to compose more and more unique functionality onto each GameObject. The result tends to be highly efficient, and therefore well-suited to a main game loop. It is also quite flexible, in that adding and removing (Mono)behaviors is simple.
In Strange, we tend to layer View and Mediator classes onto the entity for orchestration and management...but bear in mind that MVC systems, including firing Commands and accessing Services and Models, are NOT as efficient as Entity systems. Your main game loop — things that happen every frame — are probably best managed by the Entity System, whereas discrete events such as player/enemy destruction/creation, point totalling, HUD updates and so on, are properly the province of Strange.
Entity Component System - Wikipedia
Factory Pattern
The Factory is a design pattern that allows a client class to call on a third-party class (the 'factory') to provide instances of some other class. The factory can be fed with particulars that allow it to return any of one or more concrete instances. For example, by Gamefield class needs a new Enemy. I can hand the Factory some details and it can spit me out something that satisfies an IEnemy interface. This allows Gamefield to have dependencies only on IEnemy and the Factory, rather than know anything about the many Enemy subclasses (Minion, Predator, Boss) that might populate the game.
In Strange, the Factory pattern is mostly managed through the Injector: you bind an Interface to the concrete class and anyone injecting will receive it.
If your factory requires more logic than just identifying the interface, however, it may be useful to think in terms of Factory Commands. See Command Pattern above for details, and imagine that the logic for deciding what instance will be generated will live inside the Command.
Factory Method Pattern - Wikipedia
Injection
see Dependency Injection)
Inheritance
Inheritance is the principle whereby one class (the subclass) may 'extend' another (the superclass). The inheriting class essentially becomes an instance of the superclass, plus whatever new behavior the subclass adds into the mix. The inheriting subclass may also choose to override some behaviors of the superclass.
In Strange, you may bind any superclass to its subclass. Thus if a client injects the superclass, they'll receive an instance of the subclass. It is generally considered preferable to inject an interface, instead of a superclass.
Interface
An interface is a description of what a class's inputs and outputs look like, without any actual implementation of those methods.
Example:
//The interface just describes what a class might do interface ISocialService { void PostToFeed(string message); HashSet<Friend> GetFriends(); } //The implementing class does the actual work class FacebookService : ISocialService { public void PostToFeed(string message) { //work here to post the message } public HashSet<Friend> GetFriends() { //work here to get the friends list return friendSet; } }
In Strange, we can bind and inject interfaces, rather than concrete classes. Doing so allows greater flexibility. In the provided example, you could inject ISocialService anywhere you need it. If you decided later to change from Facebook to Twitter or G+, you could do so without needing to make changes throughout your application.
Model
The 'M' in MVCS. Models are objects that hold data. Basically, they keep and maintain state, usually without any logic regarding what to do with that state.
Strange has no 'Model' class. Indeed, it's best if your models have no knowledge of Strange at all. They just hold data. That's it. Finito.
Observer Pattern
A simple design pattern in which one class (the observer) watches another class (the subject) waiting for 'a thing' to happen. If the subject does that thing, the observer reacts. This is the basis of both Strange's EventDispatcher and its Signals communications systems.
Polymorphism
From the Greek meaning "many shapes", polymorphism refers to the ability of a class to represent more than one type of class or Interface. Some languages support multiple inheritance. In languages that only support single inheritance, like C#, polymorphism is achieved by implementing multiple interfaces.
For example, I can have a class like this:
class Spaceship : IVehicle, IWeapon { //implement it here }
The Spaceship above is polymorphic because at any given moment it can represent either a vehicle or a weapon. This allows extra flexibility and decoupling when writing code that interacts with the Spaceship.
Pools / Pooling
Pools are a design implementation for adding efficiency to a programming runtime. Instead of creating/destroying objects as an application requires them, a pool handles created objects and recycles them. Object creation can be expensive in terms of both memory use and performance. Object pooling can minimize these negative effects.
In Strange v0.7 we added an object pooling feature. Mostly this was added for internal efficiency, but a happy side-effect is that the pooling mechanism is available to you for whatever use you may have.
Object Pool Pattern - Wikipedia
Service
(Not to be confused with Service Locator))
Service is the 'S' in MVCS. Services represent any part of your application that handles communication outside the application itself. Calls to servers, the web, a device's native layer, a disk drive...these are all services.
Strange has no explicit 'Service' class. We use the term contextually to express the important concept of anything that reaches beyond the border of the game/app itself.
(I couldn't find a specific Wikipedia entry covering this use of 'Service' in MVCS...though there are many articles around the Internet that do so.)
Service Locator Pattern
(No relation to Service, described above)
This design pattern allows one class to locate another class via a central registry. In Strange, the central registry is the Injector. When a class injects the interface, superclass or concrete class associated with the desired injection, the Injector "locates the service" to provide runtime coupling. This avoids direct coupling and thereby makes code more flexible.
Service Locator Pattern - Wikipedia
Singleton Pattern
Singleton is a design pattern that ensures the creation of one and only one instance of a given class. It's a useful pattern in that many classes in a game or application require only a single instance. For example, you may have only one player or one communicator to your game server. But Singleton also has a number of downsides/anti-patterns associated with it: what happens if your single-player game becomes two-player? What if you change game servers or decide the split the responsibilities of the server communicator? In these and many other cases, the typical Singleton pattern of...
MySingleton.Get().DoAThing();
...creates dependency coupling that make refactoring complicated.
In Strange, instead of writing a Singleton, we map a Singleton. This means that neither the class that is the Singleton, nor the class that consumes it knows it's intended to be used that way. This gives us the 'service locator' advantage of a simple MySingleton.Get() without the concrete dependency of the specific class.
One thing Strange doesn't give you (that the Singleton pattern does) is 'Singleton enforcement'. Enforcement is the part of the pattern that armors the Singleton to ensure that no one can ever get more than one. We look at this as a good thing. Just because a class was first assumed to be a Singleton (as with a Player class) doesn't mean it will never want to be reused differently (as when your game becomes multiplayer). Indeed, Strange gives us the capability to use named instances to have multiple so-called Singletons.
View
Views are the 'V' in MVC / MVCS. Views represent anything you can see or hear, tap or click, in an application. In Unity, these are MonoBehaviours/GameObjects. Strange has a 'View' class which you can extend to allow your View to be mediated (see the Big Strange How-To section on Mediation).